Shadow Showcase
This is a demonstration of some of what you can do with shadow properties.
Drop Shadow
A drop shadow is cast by the light obstructed by an element.
Typically, the light source is directly above the element, so a drop shadow is directly below the element. This is done with a positive Y offset. You will probably want a small blur radius to give it a softer appearance. You can chose a color based on the strength of the shadow you want to portray.
.drop-shadow {
box-shadow: rgba(0, 0, 0, 0.2) 0px 4px 4px 0px;
}
Floating
To create a floating effect, you cast a light offset shadow below the element.
The code below is what Material Design uses for its floating action buttons.
.floating {
box-shadow:
0px 3px 5px -1px rgba(0, 0, 0, 0.2),
0px 6px 10px 0px rgba(0, 0, 0, 0.14),
0px 1px 18px 0px rgba(0,0,0,.12);
}
You could simplify it to a single shadow (as below) if you wish, but it won't as smooth and nuanced.
.floating {
box-shadow: 0px 6px 10px 0px rgba(0, 0, 0, 0.2),
}
Sunken
You can use a shadow to show that something is sunken into a surface. To achieve this you want to use an inner shadow - a shadow inside the content area of the element.
You use the inset
keyword with box-shadow
to create inner shadows. A subtle contrast works best most of the time. Use a slightly darker color than the background color, and a blur radius to spread and soften the shadow around the edges of the element. It creates a darker bevel really.
.sunken {
box-shadow: rgb(223, 222, 222) 0px 0px 6px 1px inset;
background-color: rgba(233, 233, 233, 0.25);
}
This example looks like a checkbox. You can use linear gradients and multiple shadows to create more nuanced effects.
Glow
To create a glowing effect, you can add multiple shadows with increasing blur radii and lighter colors.
This example is like a bright light. We use 2 shadows with no offsets. Each subsequent shadow has a larger blur radius, and has a lighter color (more transparent). This creates a diffuse effect.
.glow {
border-radius: 50%;
background-color: rgb(255, 238, 0);
box-shadow: 0 0 20px rgba(255, 230, 0, 0.8),
0 0 30px rgba(255, 230, 0, 0.6);
}
Transparent Image Shadow
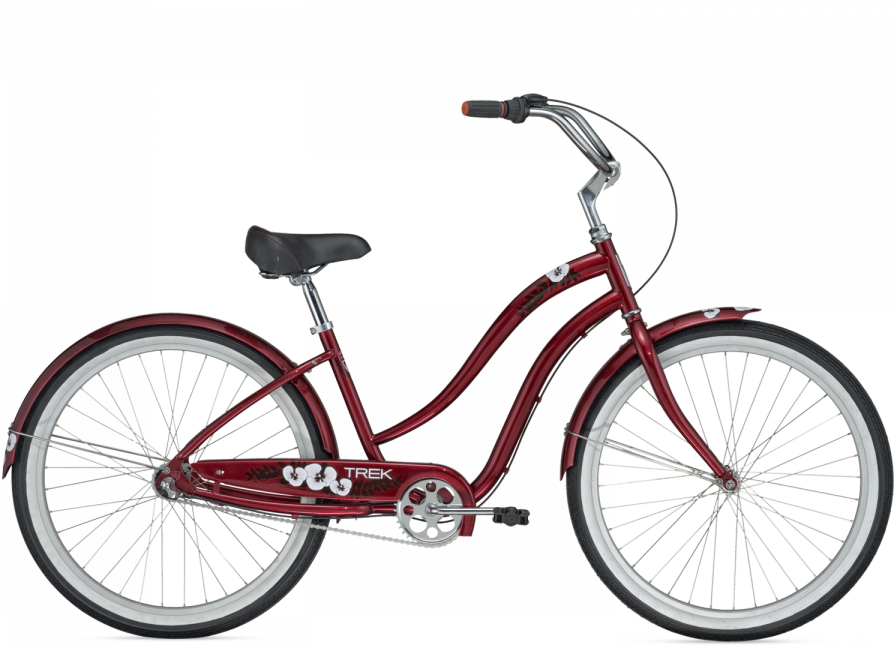
Transparent images (or more accurately, images with transparent backgrounds) can have shadows applied to highlight the outline of the visible parts. The most commonly used transparent images formats now are: GIF, PNG, WEBP, and SVG.
The drop-shadow()
filter is used to apply this type of shadow. It has a similar syntax to box-shadow
but has no spread radius.
In this example, we add a shadow to a PNG image of a bicycle.
.png-shadow {
filter: drop-shadow(20px 10px 1px rgba(0, 0, 0, 0.4));
}
Grouped Elements Shadow
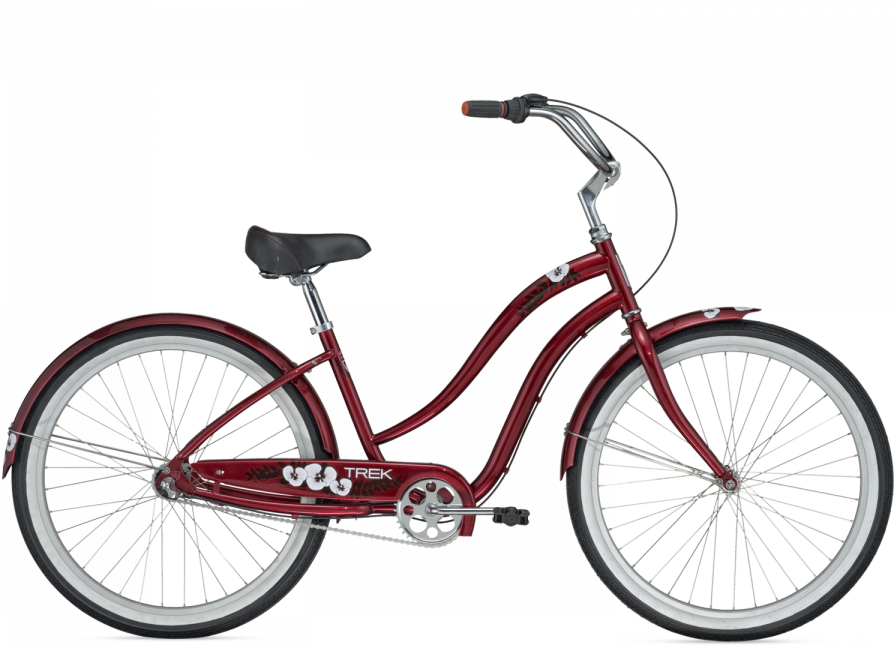
If you have a group of elements, a box-shadow
can look odd if the elements overflow the containing element. The shadow is based on the dimensions of the container only.
If you want a shadow to form around the outline of the grouped element, you can use the drop-shadow()
filter instead.
<div class="group">
<div class="rounded-rectangle"></div>
<img src="https://cdn.imgpaste.net/2021/01/12/U0oK2.png"/>
</div>
.group {
filter: drop-shadow(20px 10px 1px rgba(0, 0, 0, 0.432));
}
Mutliple Outlines
We can use box-shadow
to create as many duplicates of an element as we want. We can create them to look like outlines/borders.
We achieve this through the spread radius. We increase the spread radius for each subsequent shadow to make a new outline. In this example, each outline is 3px wide.
.multiple-outlines {
box-shadow: rgb(85, 91, 255) 0px 0px 0px 3px,
rgb(31, 193, 27) 0px 0px 0px 6px,
rgb(255, 217, 19) 0px 0px 0px 9px,
rgb(255, 156, 85) 0px 0px 0px 12px,
rgb(255, 85, 85) 0px 0px 0px 15px;
}
Text Drop Shadow
A drop shadow is cast by obstructed light.
Typically, the light source is directly above the element, so a drop shadow is directly below the element. This is done with a positive Y offset, and a small blur radius to give it a softer appearance.
The syntax for text-shadow
is similar to box-shadow
, but it does not have a spread radius.
.text-drop-shadow {
color: blue;
text-shadow: -1px 3px 2px rgba(0, 0, 0, 0.3);
}
Text "Drop Shade"
The drop shade is a technique where there is a clear separation between the text and its shadow. It can make text look like it is cut out of paper.
This can be achieved using two text-shadows — one nearer to the letterform that matches the background color, and one further away from the text that is a darker color.
.text-drop-shade {
color: blue;
text-shadow: -2px 1px 0 pink, -6px 3px 0 rgba(0, 0, 0, 0.5);
}
If the background is not a solid color (has opacity of less than 1), this won’t work as well because of color blending. You could try to compensate for this with blending modes, I guess?
3D Text
You can use text shadows to give text a thicker 3D appearance.
You can create this effect by adding a series of text shadows using small offsets (1px in this case). You can make the text shadows below the text darker than the text shadows offset more to create bevelled sides.
.text-3d-shadow {
font-family: "Alfa Slab One", sans-serif;
color: black;
text-shadow:
0 1px darkgrey,
-1px 0 lightgrey,
-1px 2px darkgrey,
-2px 1px lightgrey,
-2px 3px darkgrey,
-3px 2px lightgrey,
-3px 4px darkgrey,
-4px 3px lightgrey,
-4px 5px darkgrey,
-5px 4px lightgrey,
-5px 6px darkgrey,
-6px 5px lightgrey,
-6px 7px darkgrey,
-7px 6px lightgrey,
-7px 8px darkgrey,
-8px 7px lightgrey;
}
Queue (Vertical)
We can use box-shadow
to create many duplicates, and line them up like a queue.
By interspering larger white shadows with smaller blue shadows, we create white boxes with blue borders.
The final piece is to use a negative value for the spread radius to make the duplicates smaller. We can stagger them behind the element using a negative Y offset.
.rectangle {
background: white;
width: 100px;
height: 100px;
}
.queue-vertical {
border: 2px solid blue;
box-shadow:
white 0 -8px 0px -6px,
blue 0 -8px 0px -4px,
white 0 -16px 0px -10px,
blue 0 -16px 0px -8px,
white 0 -24px 0px -14px,
blue 0 -24px 0px -12px;
}
Queue (Offset 1)
We can use box-shadow
to create many duplicates, and line them up like a queue.
By interspering larger white shadows with smaller blue shadows, we create white boxes with blue borders.
Here we are using the X offset and Y offset to stagger them to one side.
.rectangle {
background: white;
width: 100px;
height: 100px;
}
.queue-offset1 {
border: 2px solid blue;
box-shadow:
white -10px -10px 0px -3px,
blue -10px -10px 0px -1px,
white -20px -20px 0px -3px,
blue -20px -20px 0px -1px;
}
Queue (Offset 2)
We can use box-shadow
to create many duplicates, and line them up like a queue.
By interspering larger white shadows with smaller blue shadows, we create white boxes with blue borders.
Here we are using the X offset and Y offset to stagger them to one side.
.rectangle {
background: white;
width: 100px;
height: 100px;
}
.queue-offset2 {
box-shadow:
white 10px -10px 0px -3px,
blue 10px -10px 0px -1px,
white 20px -20px 0px -3px,
blue 20px -20px 0px -1px;
}
Faux Stack (Vertical)
We can use box-shadow
to create as many duplicates of an element as we want. We can set them up to look like stacks.
In this example, we stack them vertically through positive Y offsets.
.stack-vertical {
border: 1px blue solid;
box-shadow:
rgba(59, 46, 240, 1) 0px 4px,
rgba(59, 46, 240, 0.3) 0px 7px,
rgba(59, 46, 240, 0.2) 0px 10px,
rgba(59, 46, 240, 0.1) 0px 12px;
}
Faux Stack (Offset 1)
We can use box-shadow
to create as many duplicates of an element as we want. We can set them up to look like stacks.
In this example, we stack them to the side through the X offset and Y offset.
.stack-offset1 {
border: 1px blue solid;
box-shadow:
rgba(59, 46, 240, 0.4) -5px 5px,
rgba(59, 46, 240, 0.4) -10px 10px,
rgba(59, 46, 240, 0.3) -15px 15px;
}
Faux Stack (Offset 2)
We can use box-shadow
to create as many duplicates of an element as we want. We can set them up to look like stacks.
In this example, we stack them to the side through the X offset and Y offset.
.stack-offset2 {
border: 1px blue solid;
box-shadow:
rgba(59, 46, 240, 0.4) 5px 5px,
rgba(59, 46, 240, 0.4) 10px 10px,
rgba(59, 46, 240, 0.3) 15px 15px;
}
Neumorphism (Flat)
Neumorphism is a new take on skeumorphism. It creates a soft interface with elements appearing to be placed underneath the surface of the interface.
To achieve the effect you must use a cohesive color palette. The subtle outlines for elements are created with shadows. There is a darker shadow above the element, and a lighter shadow below the element.
In this example, the element has a flat surface.
.neumorphism {
background-color: #e0e0e0;
}
.neumporphism-flat {
border-radius: 50px;
background-color: #e0e0e0;
box-shadow: 20px 20px 60px #bebebe,
-20px -20px 60px #ffffff;
}
Neumorphism (Concave)
Neumorphism is a new take on skeumorphism. It creates a soft interface with elements appearing to be placed underneath the surface of the interface.
To achieve the effect you must use a cohesive color palette. The subtle outlines for elements are created with shadows. There is a darker shadow above the element, and a lighter shadow below the element.
In this example, the element has a concave surface. A linear gradient is used to give this impression.
.neumorphism {
background-color: #e0e0e0;
}
.neumporphism-concave {
border-radius: 50px;
background: linear-gradient(145deg, #cacaca, #f0f0f0);
box-shadow:
20px 20px 60px #bebebe,
-20px -20px 60px #ffffff;
}
Neumorphism (Convex)
Neumorphism is a new take on skeumorphism. It creates a soft interface with elements appearing to be placed underneath the surface of the interface.
To achieve the effect you must use a cohesive color palette. The subtle outlines for elements are created with shadows. There is a darker shadow above the element, and a lighter shadow below the element.
In this example, the element has a concave surface. A linear gradient is used to give this impression.
.neumorphism {
background-color: #e0e0e0;
}
.neumporphism-convex {
border-radius: 50px;
background: linear-gradient(145deg, #f0f0f0, #cacaca);
box-shadow:
20px 20px 60px #bebebe,
-20px -20px 60px #ffffff;
}
Neumorphism (Pressed)
Neumorphism is a new take on skeumorphism. It creates a soft interface with elements appearing to be placed underneath the surface of the interface.
To achieve the effect you must use a cohesive color palette. The subtle outlines for elements are created with shadows. There is a darker shadow above the element, and a lighter shadow below the element.
In this example, the element has looks like it is pressed. Inset shadows are used to give this impression.
.neumorphism {
background-color: #e0e0e0;
}
.neumporphism-pressed {
border-radius: 50px;
background: #e0e0e0;
box-shadow:
inset 20px 20px 60px #bebebe,
inset -20px -20px 60px #ffffff;
}
Vignette
A vignette is a gradual dark shade surrounding the edges of a frame. It is used mostly for images.
We can do this with 2 inset shadows. To do it specifically with an image, don't apply it directly to an img
element as it will be hidden underneath the image. It can be applied to an element with a background image, or to a div
containing the image. I go for the former here.
You could use a radial-gradient()
, or a mask
instead for the same outcome.
.vignette {
background-image: url(img/violin.jpg);
background-size: contain;
box-shadow:
rgba(50, 50, 93, 0.75) 0px 30px 60px -12px inset,
rgba(0, 0, 0, 0.8) 0px 18px 36px -18px inset;
}
Target
Overlapping shadows can be used to hide sections of a shadow and create different shapes.
In this case, we have a stack of shadows. At the bottom is a large red shadow. We then place 4 smaller white shadows on top, offset in each direction. This will cover the majority of the red shadow and only leave small sections by the corners.
.target {
background-color: white;
box-shadow:
0px 10px 0px -4px white,
0px -10px 0px -4px white,
10px 0px 0px -4px white,
-10px 0px 0px -4px white,
0px 0px 0px 5px red;
}
CSS Art
You can create elaborate art with shadows. Having lots of shadows is impractical to maintain for regular webpages. For art projects, you can do what you want!
This eye is a single div
. As you can see from the code excerpt, it is created through box-shadow
and border-radius
only!
The shape of a shadow is based on the element it is applied to, but you can create variations by overlapping shadows. In this case,the cresent-shaped eyelid here is an example of this.
.eye {
border-radius: 50%;
box-shadow:
0 70px 0 -40px rgb(53, 32, 0) inset,
0 0 0 20px white inset,
0 0 0 25px rgb(40, 173, 0) inset,
0 0 0 40px #83d872 inset,
0 0 0 49px rgb(12, 12, 12) inset,
0 0 0 50px rgba(255, 255, 255) inset,
0 0px 0 2px rgb(53, 32, 0),
0 0 0 10px rgb(75, 45, 0);
}
SVG Path Shadow
In a SVG, you may want to have shadows for individual paths.
The <feDropShadow>
filter can be used for this purpose. It has no spread radius.
In this example, the shadow is applied to the shoe on the left shoe only.
<svg id="svg-shadow" viewBox="0 0 1200 800">
<defs>
<filter id="shadow">
<feDropShadow dx="10" dy="10"
stdDeviation="20" flood-color="black"
flood-opacity="0.75"/>
</filter>
</defs>
<path id="shoe1"
d="M351.943 571.514s-134.763 2.177-151.159 0c-16.396-2.177-62.753-40.815-62.753-40.815 0-33.219 21.633-70.213 26.961-82.682 18.27 5.542 48.504 11.525 88.787 8.495 1.02 11.433 7.14 51.625 42.627 56.329 58.78 7.734 55.537 58.673 55.537 58.673z"
class="shoe path-shadow"/>
<path id="shoe2"
d="M1164.701 578.595s-174.482 0-256.525-.499c-69.003-.445-205.73 5.56-207.008-27.635-1.196-30.581 29.748-110.122 34.53-136.95a332.979 332.979 0 00157.66-34.474c17.793 17.376 116.961 111.04 198.42 120.63 89.856 10.593 72.923 78.928 72.923 78.928z"
class="shoe"/>
</svg>
.path-shadow {
filter: url(#shadow);
}
SVG filters are not supported in Safari. So, you should consider creating a shadow manually (with another path/shape), if you want to support Safari.